TypeScript Path Aliases: Cleaner Imports for Scalable Codebases
Preface
This post covers:
- How to configure path aliases in a TypeScript project.
- The benefits of using path aliases.
- A practical example with a
tsconfig.json
configuration.
Solution
In TypeScript projects, managing long and complex import paths can become cumbersome. Instead of:
import MyComponent from "../../components/MyComponent";
import { someUtil } from "../../utils/helpers";
You can configure path aliases in your tsconfig.json
file to make imports cleaner:
{
"compilerOptions": {
"baseUrl": ".",
"paths": {
"@components/*": ["src/components/*"],
"@utils/*": ["src/utils/*"],
"@types/*": ["src/types/*"],
"@src/*": ["src/*"]
}
}
}
With this setup, you can now write:
import MyComponent from "@components/MyComponent";
import { someUtil } from "@utils/helpers";
Steps to Set Up:
- Modify
tsconfig.json
and add thepaths
configuration. - Ensure your project’s module resolution supports aliases (
moduleResolution: "node"
). - If using ES modules, update your
tsconfig.json
with:"module": "NodeNext", "moduleResolution": "NodeNext"
- If using a bundler like Webpack or Vite, configure aliases there too.
Why?
- Readability: Improves code clarity by removing unnecessary
../
paths. - Maintainability: Easier to refactor without breaking long relative paths.
- Scalability: Simplifies working on large codebases with deeply nested folders.
By setting up path aliases, you make your TypeScript project more modular, organised, and easier to navigate.
My Technical Skills
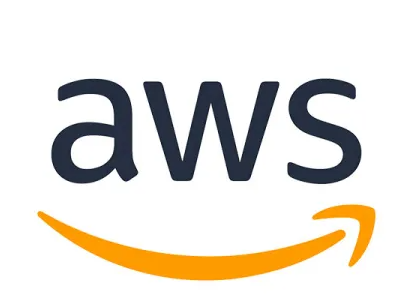
AWS
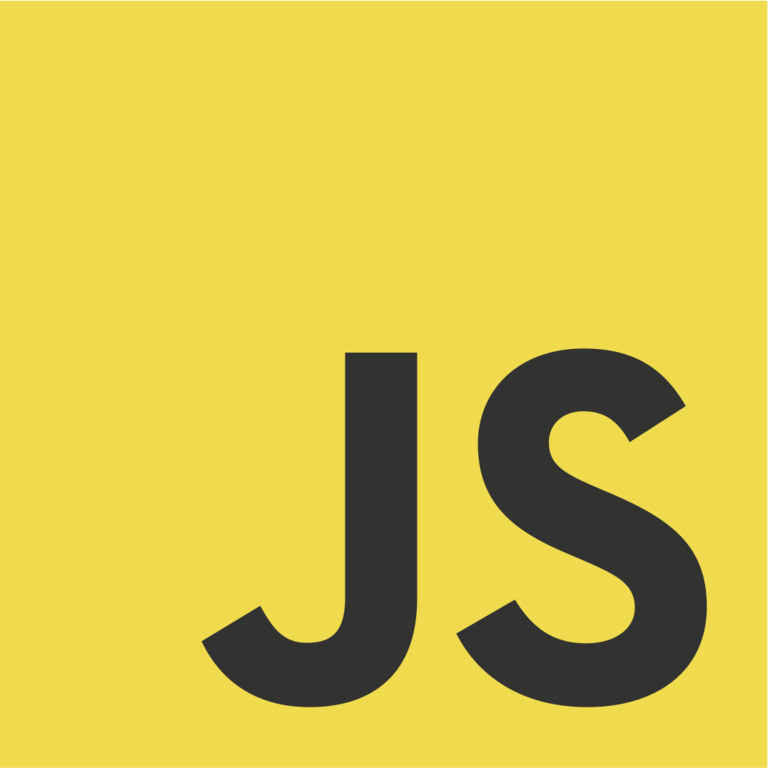
JavaScript
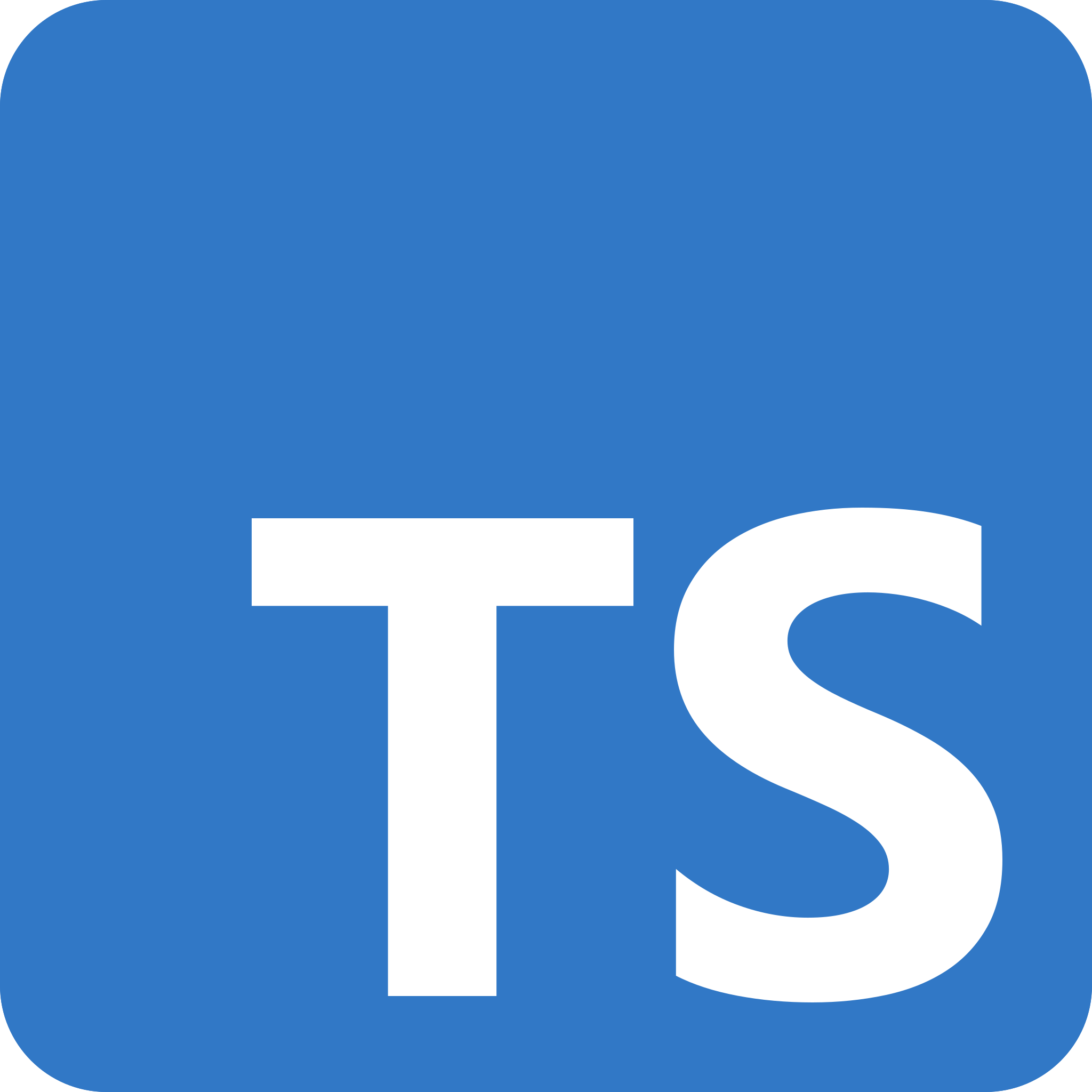
TypeScript
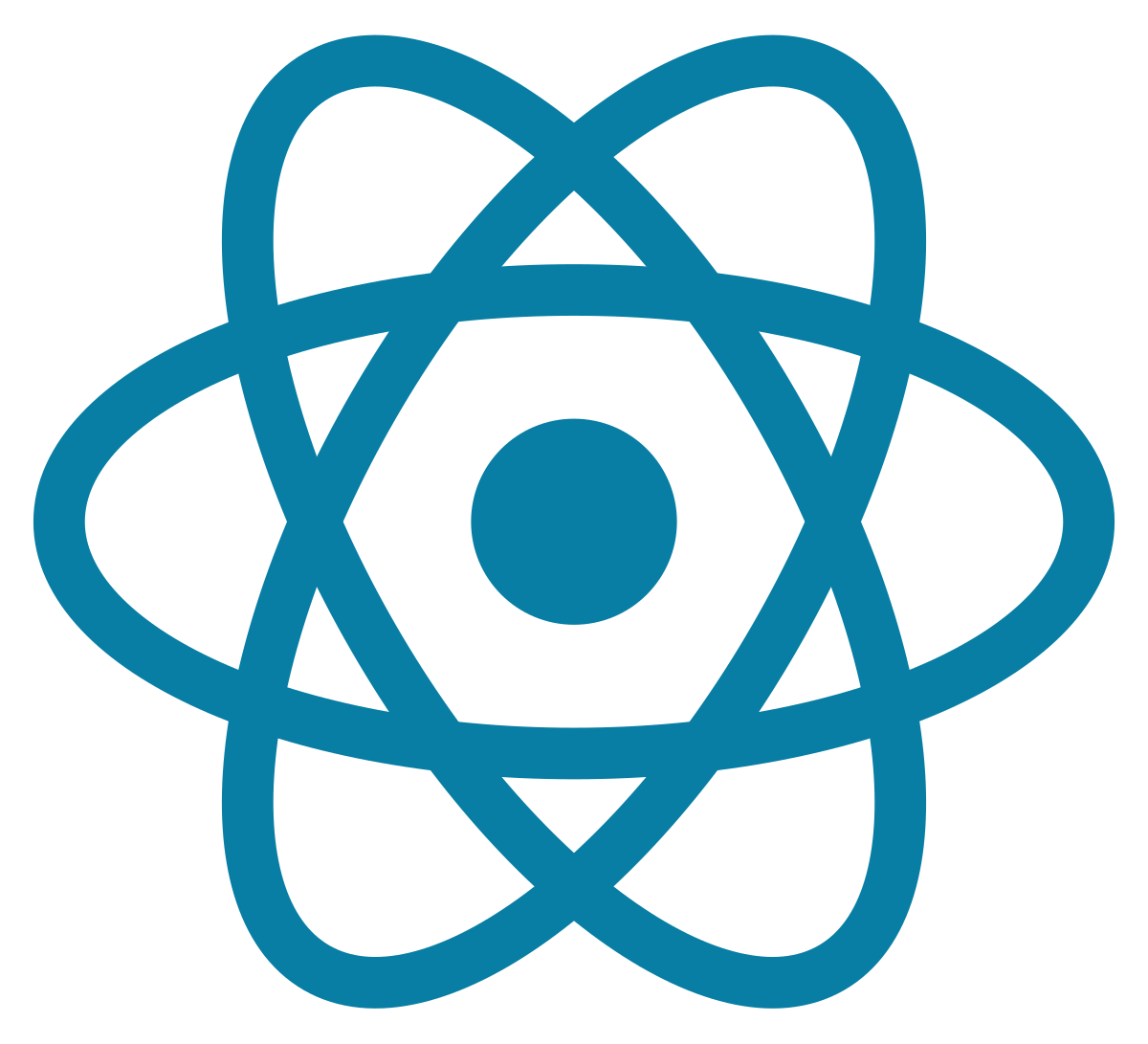
React
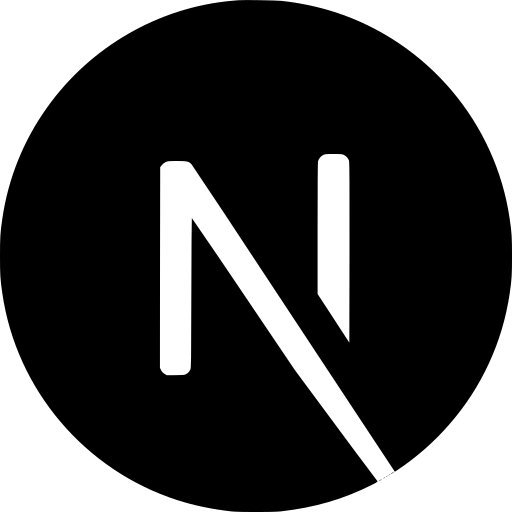
Next.js
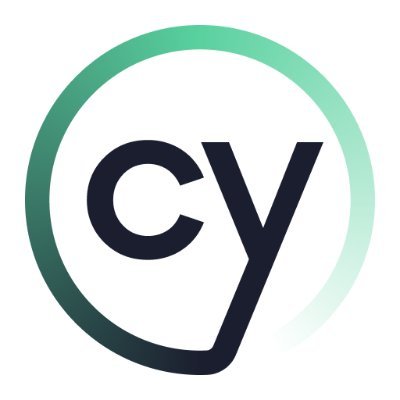
Cypress

Figma
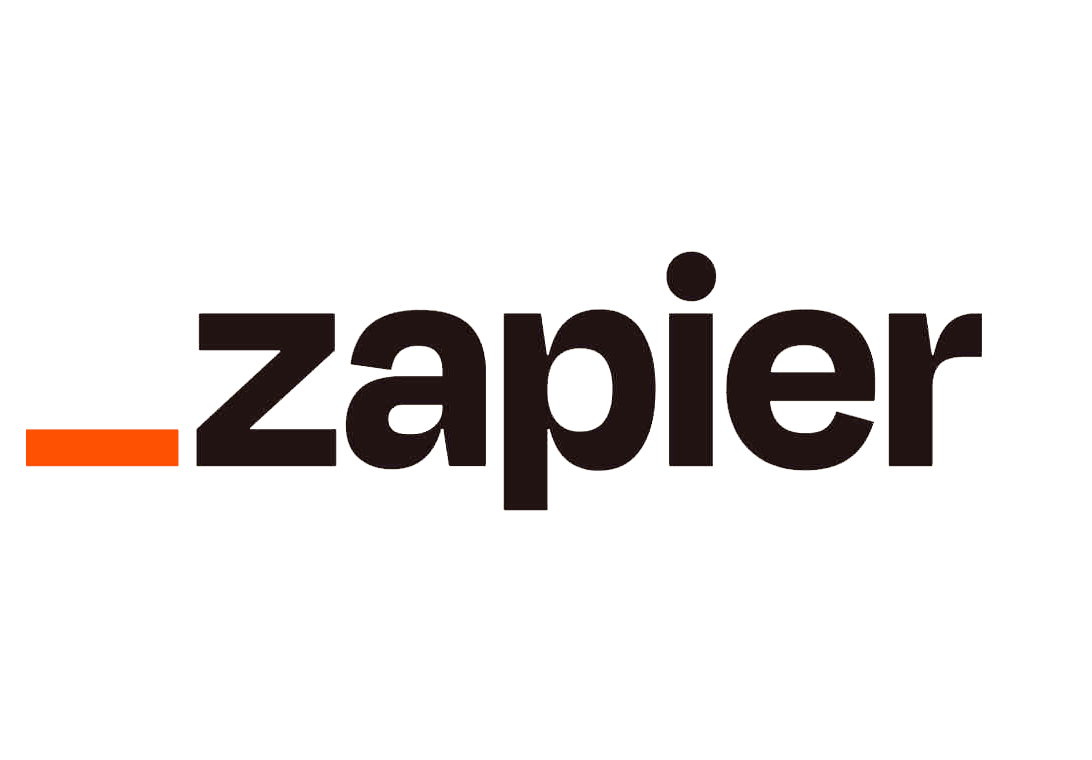