Simplifying Image Uploads in React with Cypress
In this blog post, we'll explore how to efficiently test image uploads in React applications using Cypress, a leading front-end automation testing tool. By the end of this guide, you'll understand how to overcome common testing challenges and ensure a seamless user experience for file uploads.
What You'll Learn:
- The challenge of testing hidden inputs in file uploads
- How to build an image upload button with Material-UI
- How to implement and automate image upload tests with Cypress
Understanding the Challenge: Hidden Input Fields
When developing web applications, you might encounter issues when trying to test an image upload feature, especially when the input field is hidden.
This is a common scenario in React applications, particularly when using Material-UI or similar UI libraries for styling and component abstraction. Many UI frameworks use hidden input fields wrapped inside a label to provide a better UX, but this can make testing more challenging.
Building an Image Upload Button with Material-UI
Material-UI provides a collection of pre-styled UI components that streamline front-end development. A common approach to implementing an image upload button in React + Material-UI looks like this:
<Button
datacy="uploadImage"
component="label"
variant="text"
>
<input
type="file"
onChange={(e) => onChange(e)}
hidden
accept="image/png, image/jpeg"
/>
{hasProfilePicture ? "Change" : "Upload"}
</Button>
Why Is This an Issue?
Since the <input>
field is hidden, Cypress cannot directly interact with it. This means traditional methods like .attachFile()
won’t work. Instead, we need a workaround.
The Cypress Solution: Testing Hidden File Inputs
Cypress is an end-to-end testing tool that can simulate user interactions in a web application. The key to testing hidden file inputs is targeting the label component instead of the input field.
Step-by-Step Cypress Test for Image Uploads
Here’s how you can automate an image upload test in Cypress:
// Step 1: Trigger the file selection dialog
cy.get('[datacy="uploadImage"]')
.selectFile('cypress/fixtures/example.png', { force: true });
// Step 2: Verify the file upload
cy.get('[datacy="uploadImage"] input')
.should('have.prop', 'files')
.its('length')
.should('eq', 1) // Ensure a file has been selected
.its('0.name')
.should('eq', 'example.png'); // Verify the correct file is uploaded
Why This Works:
- Targeting the label: Since Cypress can’t interact with a hidden
<input>
, we click on the wrapping label. - Using
selectFile()
: This method directly sets a file in the input field, bypassing UI restrictions. - Verifying the file selection: We check that the file has been correctly attached by asserting its
name
and length.
Implementing This in Your Application
By following this method, you can:
✔️ Ensure reliable image uploads in your React app
✔️ Automate the testing process with Cypress
✔️ Maintain a smooth user experience while improving code quality
Key Takeaways
âś… Hidden inputs can make testing file uploads tricky, but Cypress provides a way to simulate user actions effectively.
âś… Targeting the label instead of the input element is the best approach for testing Material-UI upload buttons.
✅ Cypress’ selectFile()
function allows you to accurately validate file uploads without complex workarounds.
By integrating these testing techniques into your development workflow, you can ensure a seamless image upload experience for your users while catching potential issues before they reach production.
My Technical Skills
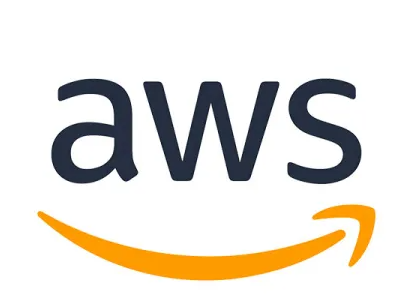
AWS
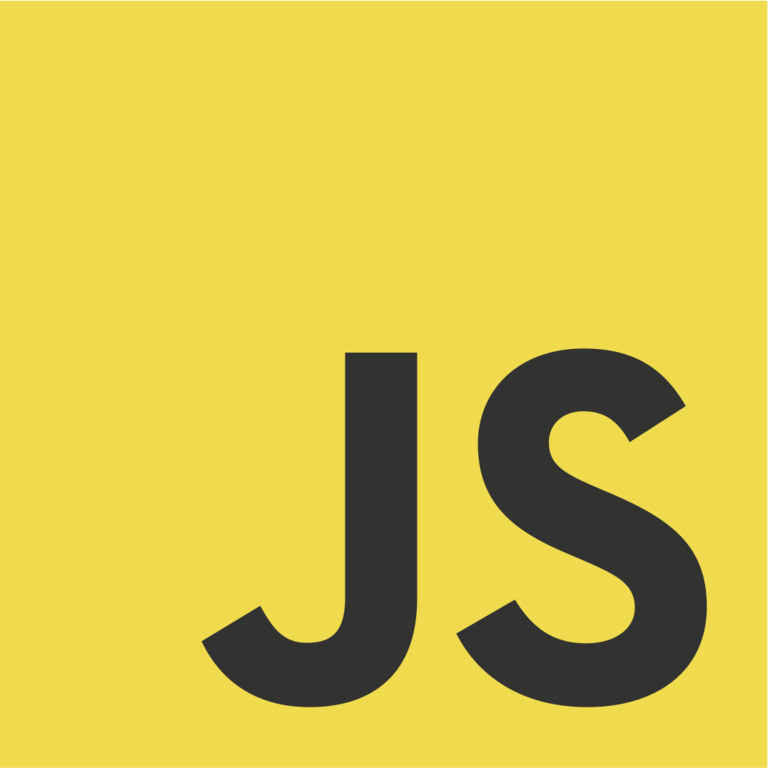
JavaScript
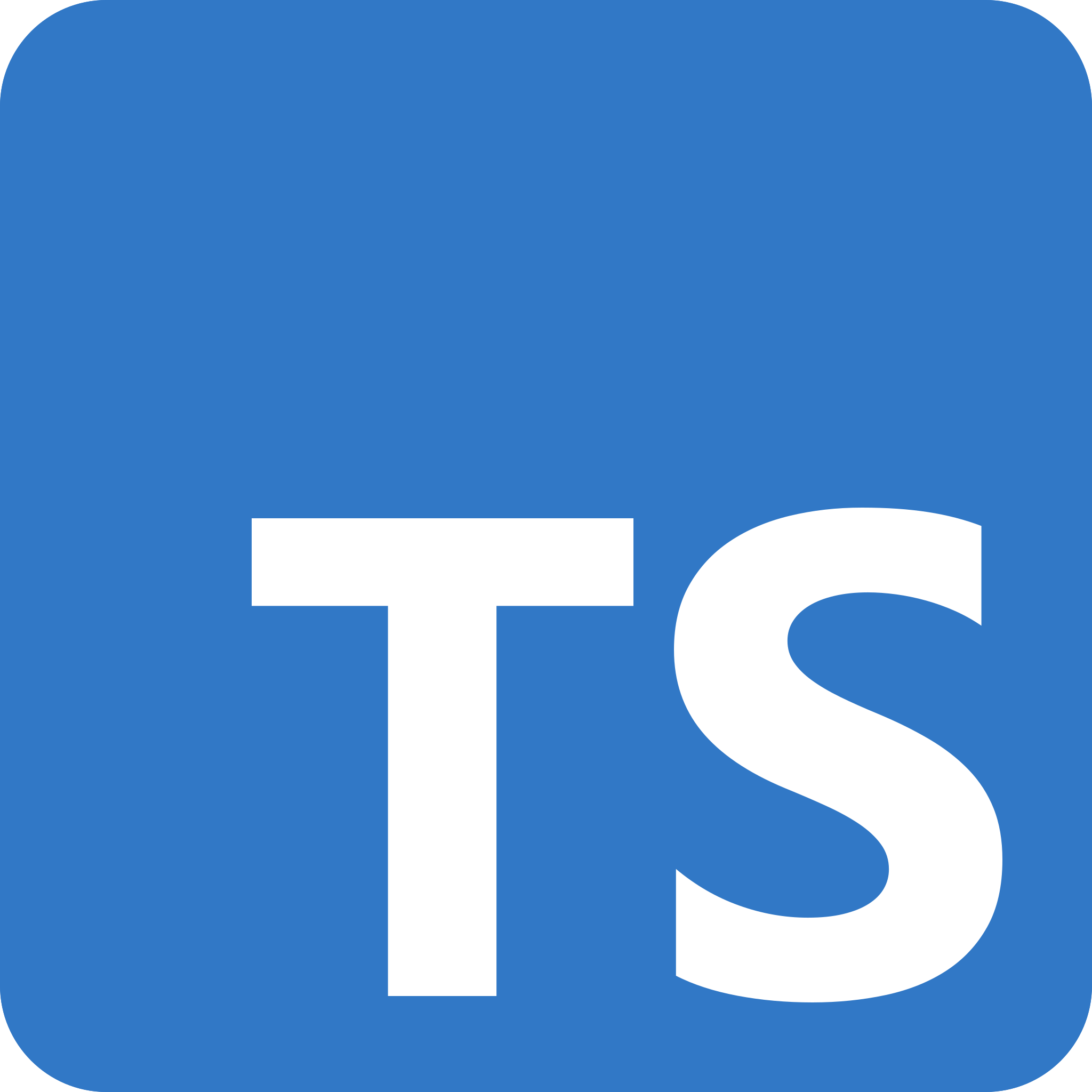
TypeScript
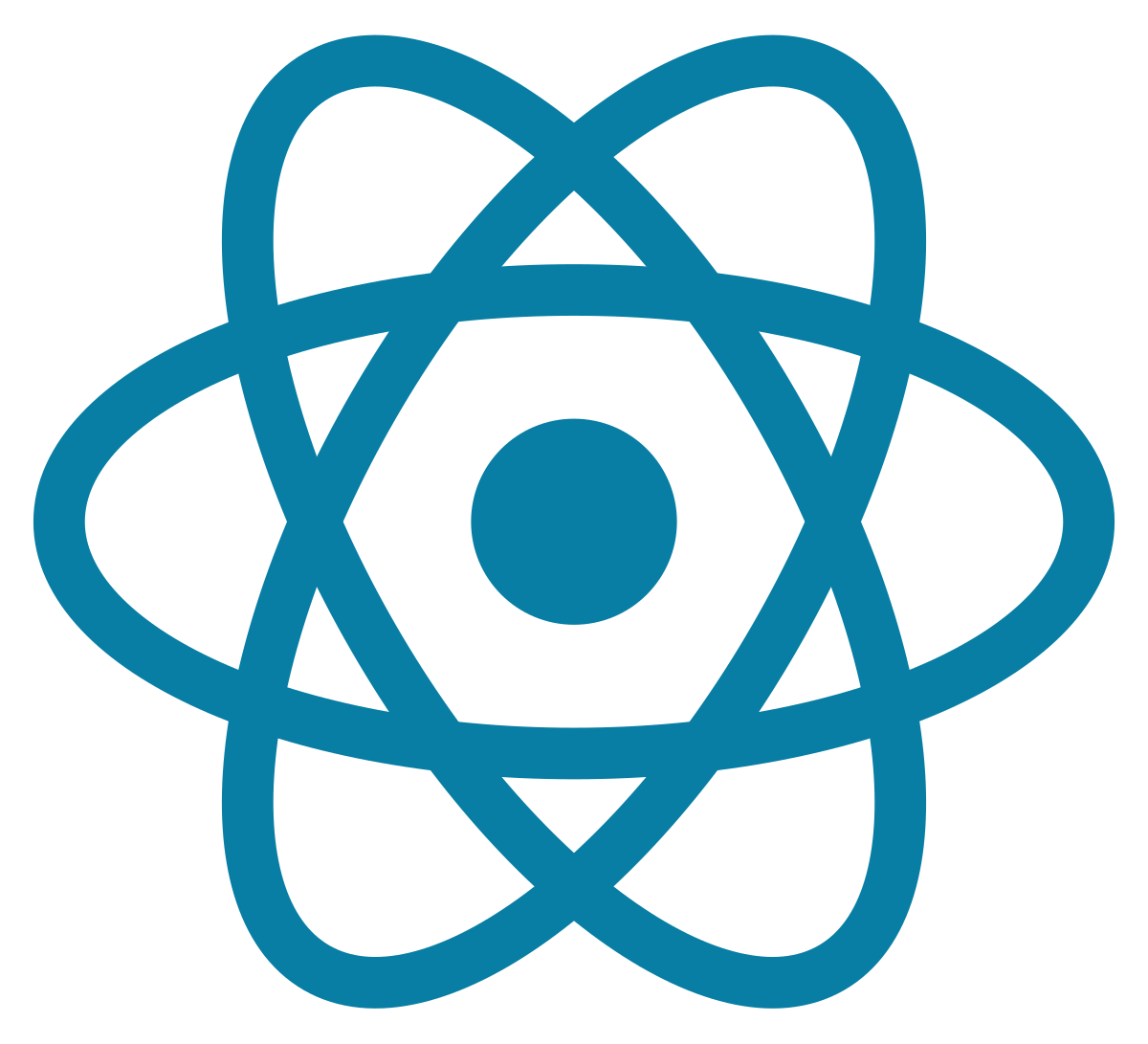
React
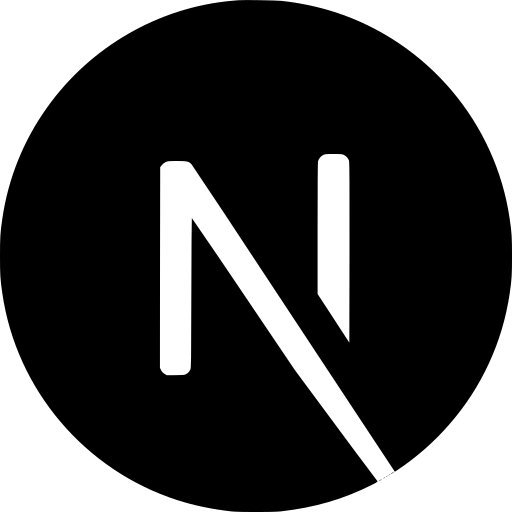
Next.js
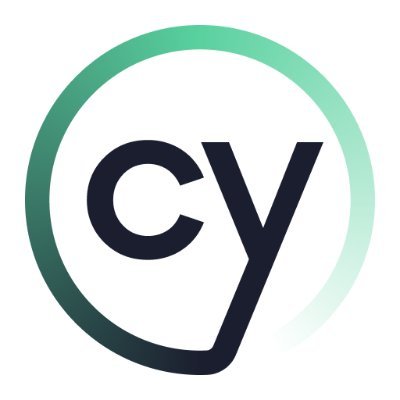
Cypress

Figma
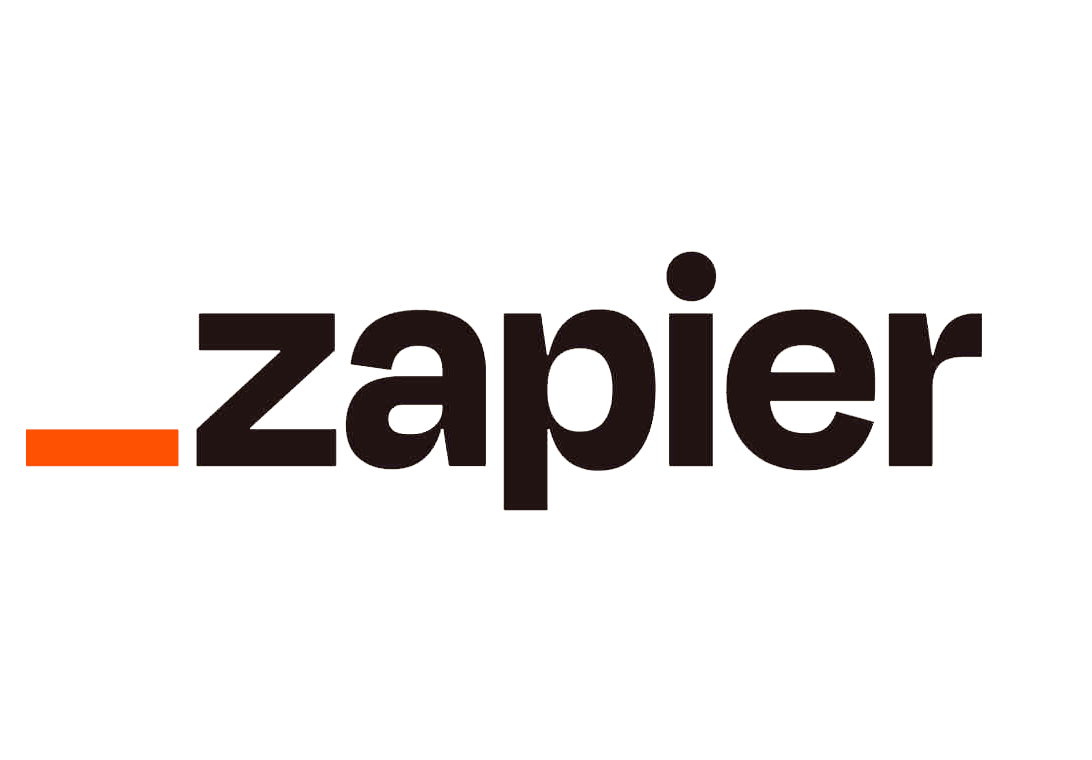